In this article I shall demonstrate an method utilising PowerShell to post messages to a Mattermost instance by means of a incoming webhook. Additionally I expanded on this further to include a working example on how you can also use the function as part of a scheduled task running on Windows.
Table of Contents
There is a Python 3 alternative to this script available; read more on how to post messages from Python 3.
Below is the function that we will be utilising to send messages to Mattermost using PowerShell.
function Post-Mattermost {
<#
.Synopsis
Post text to a Mattermost Channel via Webhooks
.DESCRIPTION
Long description
.EXAMPLE
Post-Mattermost -uri "https:///hooks/xxxxxxxxxxxxxxxxx" -text "New message from Powershell" -user "Anomandaris"
.INPUTS
$uri,$text,$user
.NOTES
Webhook url and message body are mandatory, username is optional.
#>
[CmdletBinding()]Param
(
# Incoming Webhook
[Parameter(Mandatory=$true
)]
[string]$uri,
# Body of message
[Parameter(Mandatory=$true
)]
[string]$text,
# Username to post as
[Parameter(Mandatory=$false
)]
[string]$user
)
$Payload = @{
text=$text;
username=$user;
}
Invoke-RestMethod -Uri $uri -Method Post -ContentType 'application/json' -Body (ConvertTo-Json $Payload)
}
In the example above we can see that this function Post-Mattermost
has 3 arguments, lets review them below:
Argument | Description |
---|---|
uri (required) | A url, example: https://server-url.com/hooks/xxxxxxxxxxxxxxxxx The value should be that of the incoming webhook you have generated on your server. |
text (required) | A message, example: Test message sent from PowerShell |
user | A name, example: Anomandaris |
Post-Mattermost
argument explanation.This function can be used in a few ways; below are some examples on how to call the function from PowerShell and pass in additional data.
Send a message to a webhook with a custom username:
Post-Mattermost -uri "https://myserver.com/hooks/xxxxxxxxxxxxxxxxx" -text "New message from Powershell" -user "Anomandaris"
Send a message to a webhook; will use the default username associate to the service webhook:
Post-Mattermost -uri "https://myserver.com/hooks/xxxxxxxxxxxxxxxxx" -text "New message from Powershell"
The file linked above also demonstrates how to use this function from a PowerShell script which accepts arguments and can be ran on Windows as a scheduled task.
Deploying the script as a scheduled task
Next we will look to utilise our script and function as a scheduled task. In this example our script will be triggered to run on certain logon events compatible with Windows based device (I think Windows 7 onward).
There are several user logon related scheduled task triggers that will be used in this example. Each task has two main parts; a trigger and an action (though they could have one or many). In the table below I have listed several triggers and there associated actions. You will need to create a new task for each entry in the table; updating the placeholder parameters to match your configuration and environment.
Scheduled Task | Trigger | Description | Action |
---|---|---|---|
Task 1 | On connection to user session | On remote connection to any user session | Program:powershell Add arguments: -file .\logon.ps1 -EventType "Remote Login" -WebHook "https://<webhook_url>/hooks/xxxxxxxxxxxxxxxxx" Start in: C:\<path_to_script>\ |
Task 2 | On workstation unlock | On work station unlock of any user | Program:powershell Add arguments: -file .\logon.ps1 -EventType "Workstation unlock" -WebHook "https://<webhook_url>/hooks/xxxxxxxxxxxxxxxxx" Start in: C:\<path_to_script>\ |
Task 3 | On disconnect from user session | On disconnect from any user session | Program:powershell Add arguments: -file .\logon.ps1 -EventType "Remote Logoff" -WebHook "https://<webhook_url>/hooks/xxxxxxxxxxxxxxxxx" Start in: C:\<path_to_script>\ |
Configuring the tasks trigger
Next we need to configure the trigger on the scheduled task; this is straight forward, for each task shown in the table above create and configure the triggers. As you create each task and the associated trigger check that the “Begin the task” parameter matches that of the trigger column in the table above.
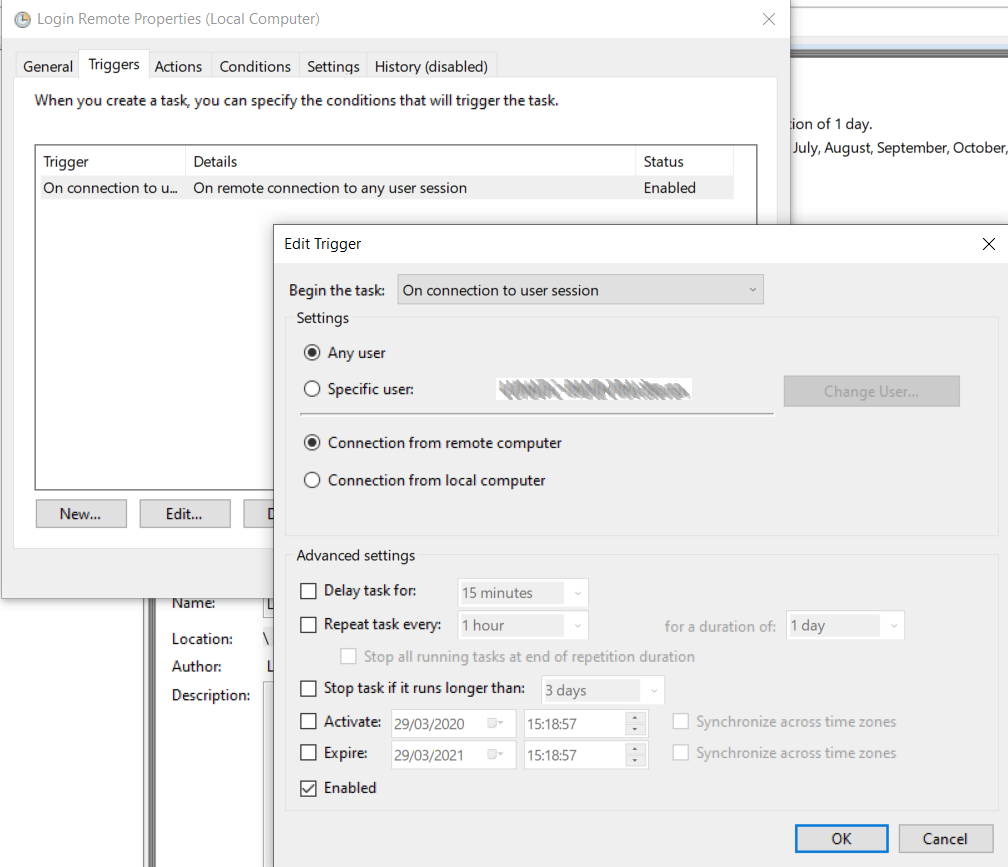
Configure the tasks action
Use the table above to set the values for arguments for fields in the action section: Program/script, Add arguments and Start in; it should look something like below:
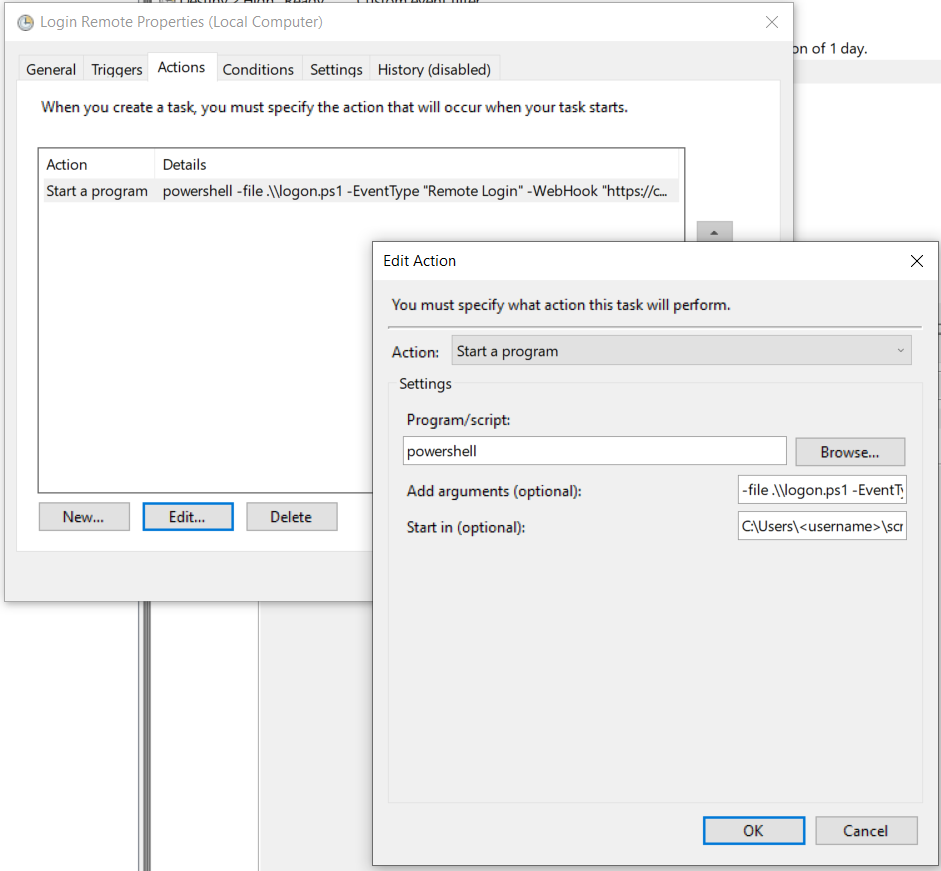
Additional information
The webhooks used by Mattermost and Slack are somewhat similar in terms of payload for basic messages. For more advanced requirements consult the relevant documentation:
- Mattermost webhooks: https://docs.mattermost.com/developer/webhooks-incoming.html
- Slack webhooks : https://api.slack.com/messaging/webhooks
- Microsoft Teams webhooks: https://docs.microsoft.com/en-us/microsoftteams/platform/webhooks-and-connectors/how-to/connectors-using#setting-up-a-custom-incoming-webhook
Now depending upon which service you are using; Mattermost or Slack you may be required to adjust the contents of the payload sent to the server. To do this find the section below and adjusting the contents to suit your needs.
$Payload = @{
text=$text;
username=$user;
}
It’s a pity you don’t have a donate button! I’d definitely donate
to this excellent blog! I guess for now i’ll settle for bookmarking and adding your RSS feed to my Google account.
I look forward to fresh updates and will share this website
with my Facebook group. Talk soon!
Thank you for the feedback, I’m glad you liked the content and look forward to seeing you round here in the future.
Peculiar article, totally what I was looking for.